yahia-elsayed
_about-me
professional-info
skills
experience
interships
personal-info
bio
interests
education
professional-info
skills
experience
interships
contact
+20 1278985786
//about-me
/ front-end
front-end
1/*
2Front-End Technologies:
3 HTML
4 CSS
5 JavaScript
6 TypeScript
7 React.js
8 Next.js
9 Responsive Design
10 Cross-Browser Compatibility
11 RESTful APIs
12 Component-Based Architecture.
13 Tailwind CSS
14 Next UI
15 Ant Design
16 Shadcn UI
17 */
// Code snippet showcase:
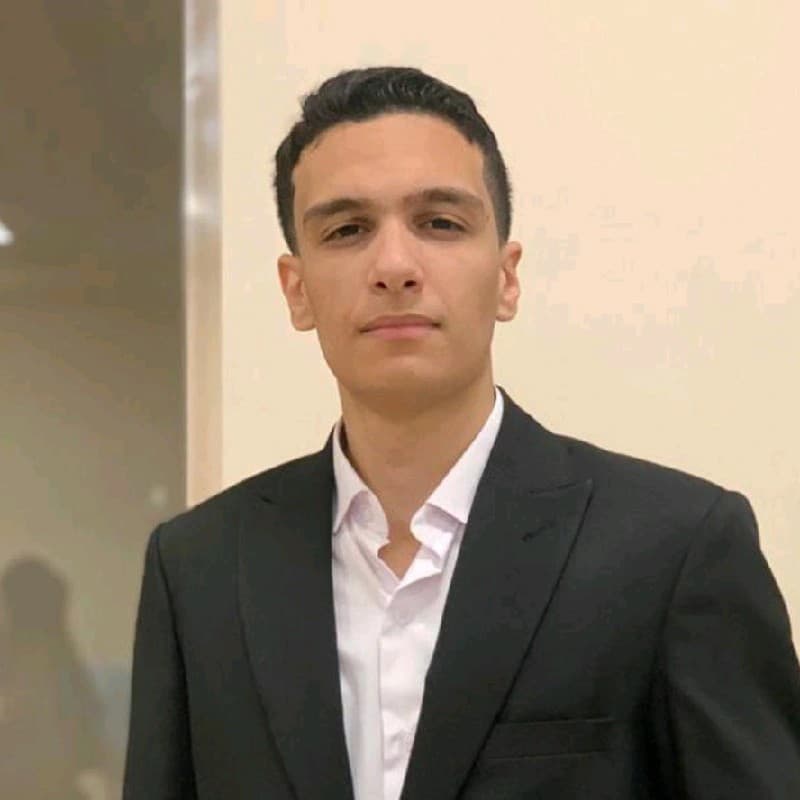
@yahiaelsayed19
created 4 months ago
import Link from 'next/link';
export default function Home() {
return (
<div>
<h1>Welcome to Next.js!</h1>
<Link href="/about">Go to About Page</Link>
</div>
);
}
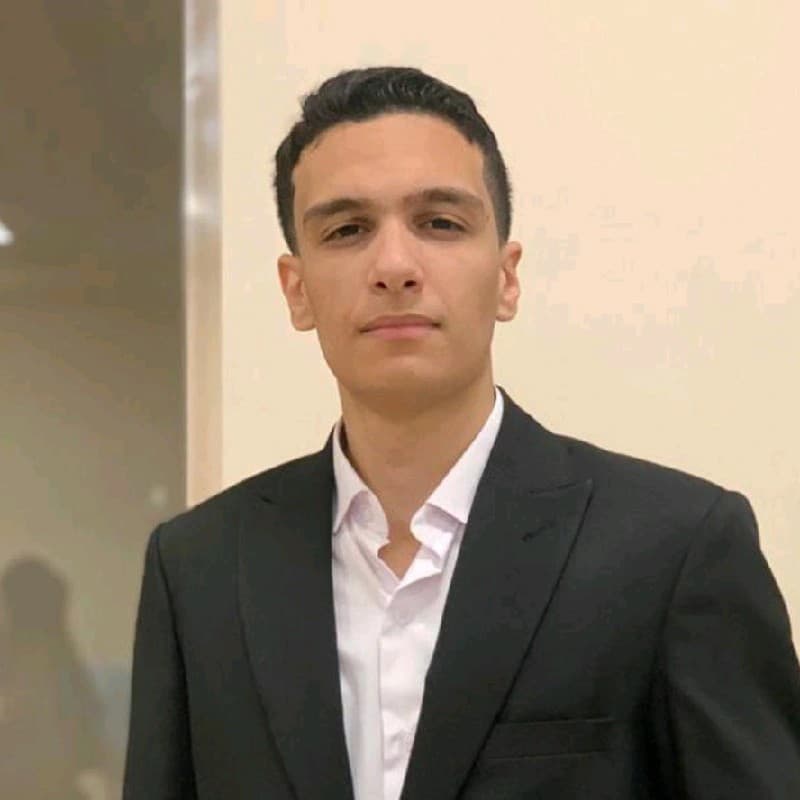
@yahiaelsayed19
created 4 months ago
import { useRouter } from 'next/router';
export default function Post() {
const router = useRouter();
const { id } = router.query;
return <p>Post ID: {id}</p>;
}
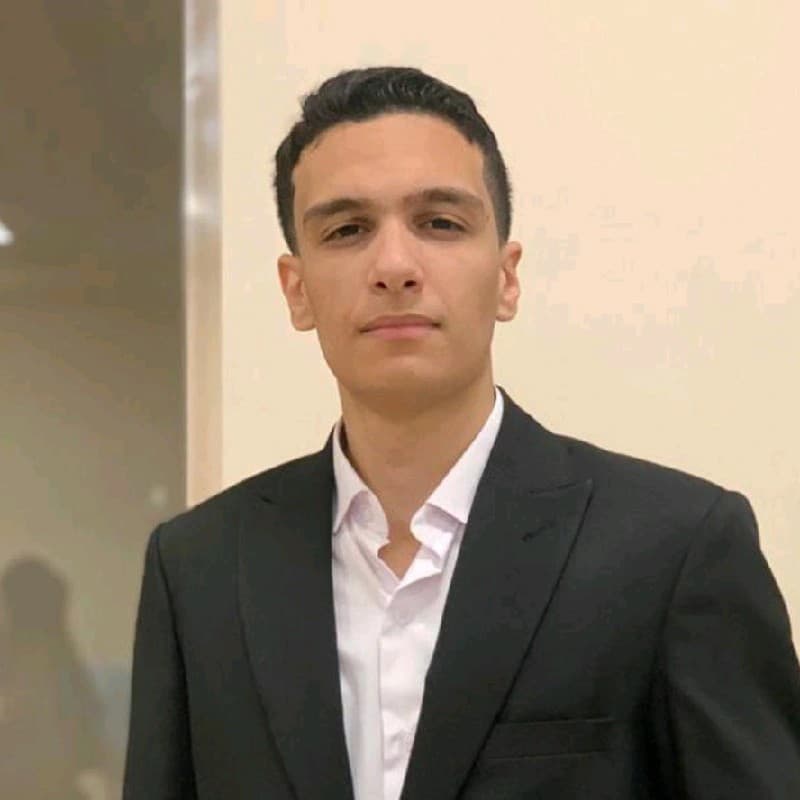
@yahiaelsayed19
created 4 months ago
import Head from 'next/head';
export default function Home() {
return (
<div>
<Head>
<title>Home Page</title>
</Head>
<h1>Welcome to Next.js!</h1>
</div>
);
}
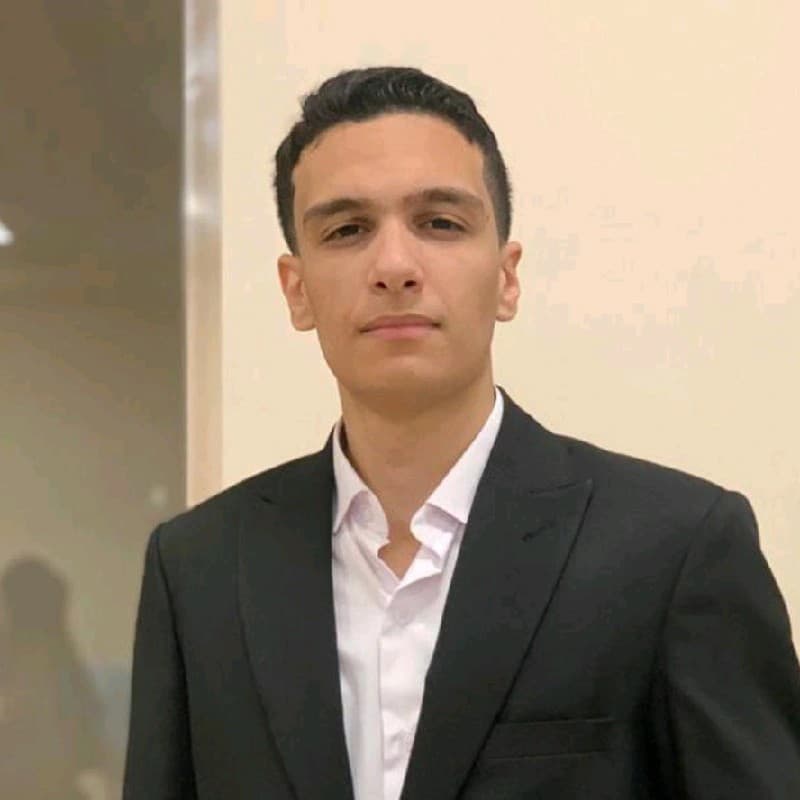
@yahiaelsayed19
created NaN years ago
import { useEffect } from 'react';
export default function Home() {
useEffect(() => {
console.log('Component mounted');
}, []);
return <h1>Welcome to Next.js!</h1>;
}
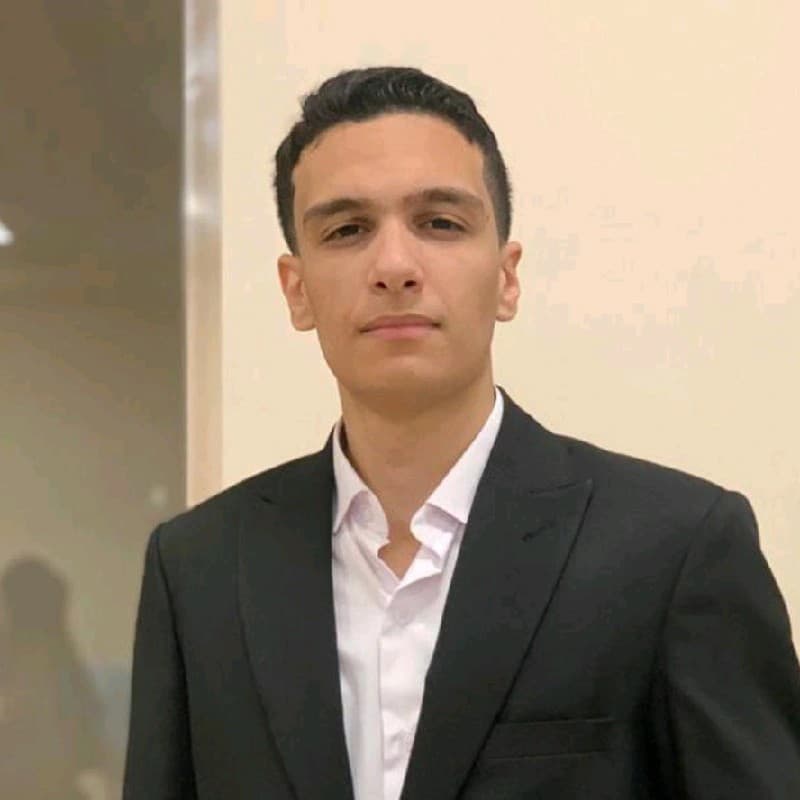
@yahiaelsayed19
created 4 months ago
import Image from 'next/image';
export default function Home() {
return (
<div>
<Image src="/vercel.svg" alt="Vercel Logo" width={72} height={16} />
<h1>Welcome to Next.js with Image Optimization!</h1>
</div>
);
}
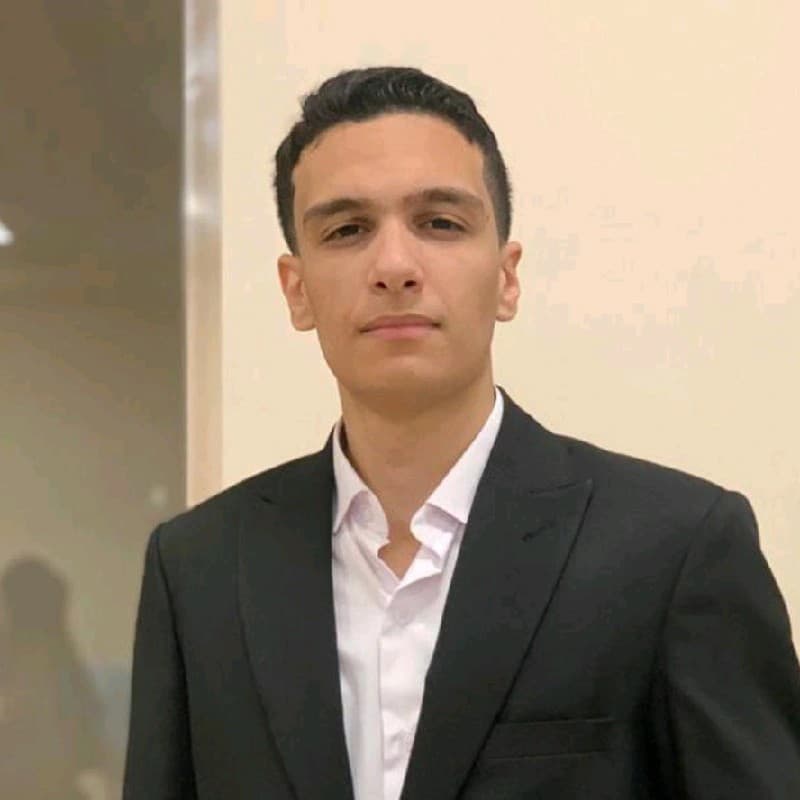
@yahiaelsayed19
created 4 months ago
export async function getStaticProps() {
return {
props: {
message: 'Hello from static props!',
},
};
}
export default function Home({ message }) {
return <h1>{message}</h1>;
}
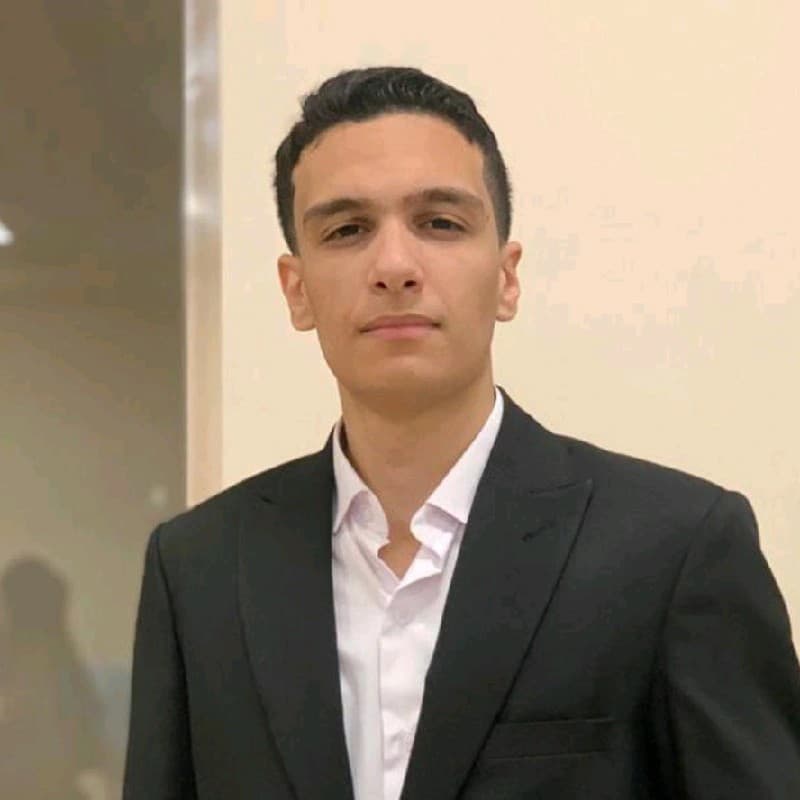
@yahiaelsayed19
created 4 months ago
export default function Custom404() {
return <h1>404 - Page Not Found</h1>;
}
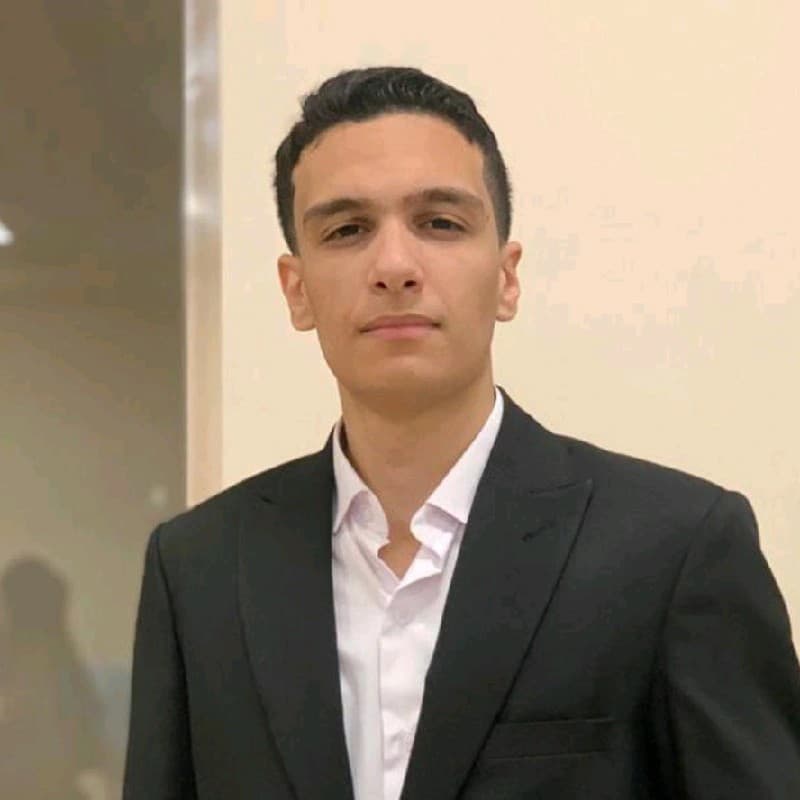
@yahiaelsayed19
created 4 months ago
import { useState } from 'react';
export default function Home() {
const [count, setCount] = useState(0);
return (
<div>
<h1>Count: {count}</h1>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
}
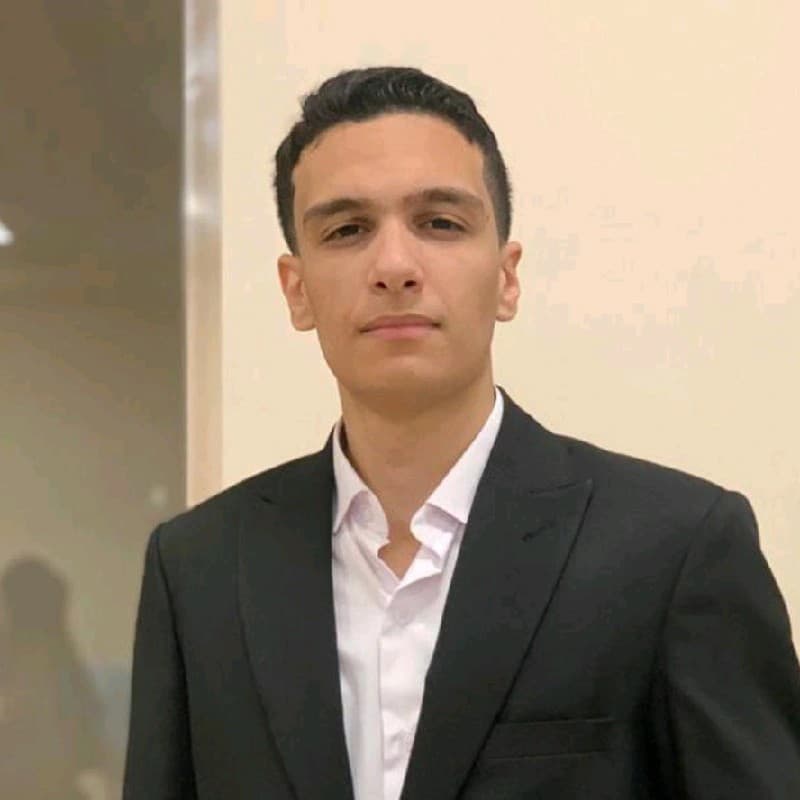
@yahiaelsayed19
created 4 months ago
import { useRouter } from 'next/router';
export default function DynamicRoute() {
const router = useRouter();
const { slug } = router.query;
return <h1>Dynamic Route: {slug}</h1>;
}
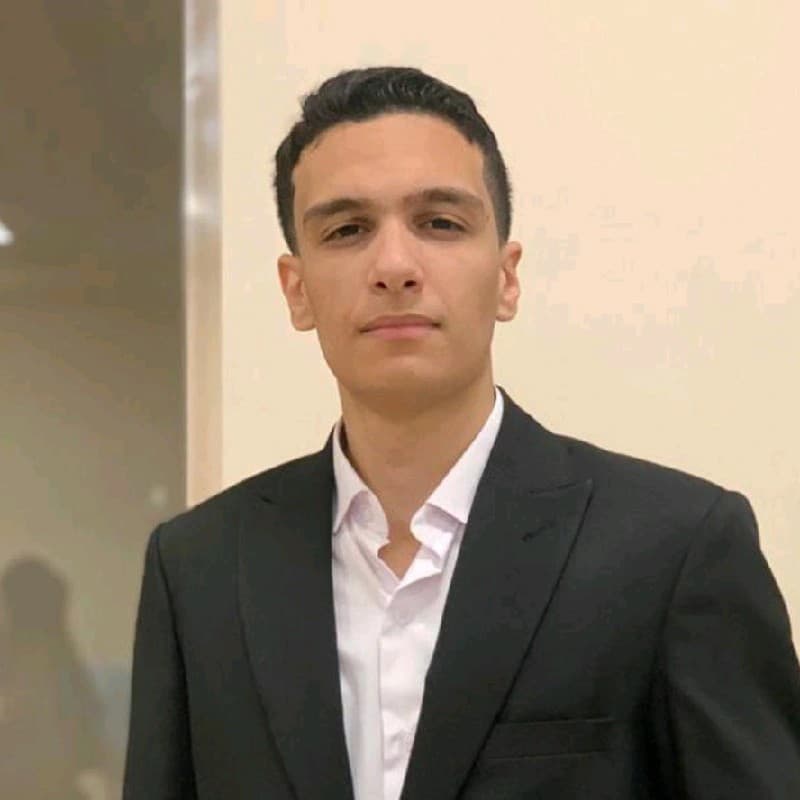
@yahiaelsayed19
created 4 months ago
import Head from 'next/head';
export default function SEO() {
return (
<Head>
<title>Next.js SEO</title>
<meta name="description" content="Learn Next.js SEO optimization" />
</Head>
);
}
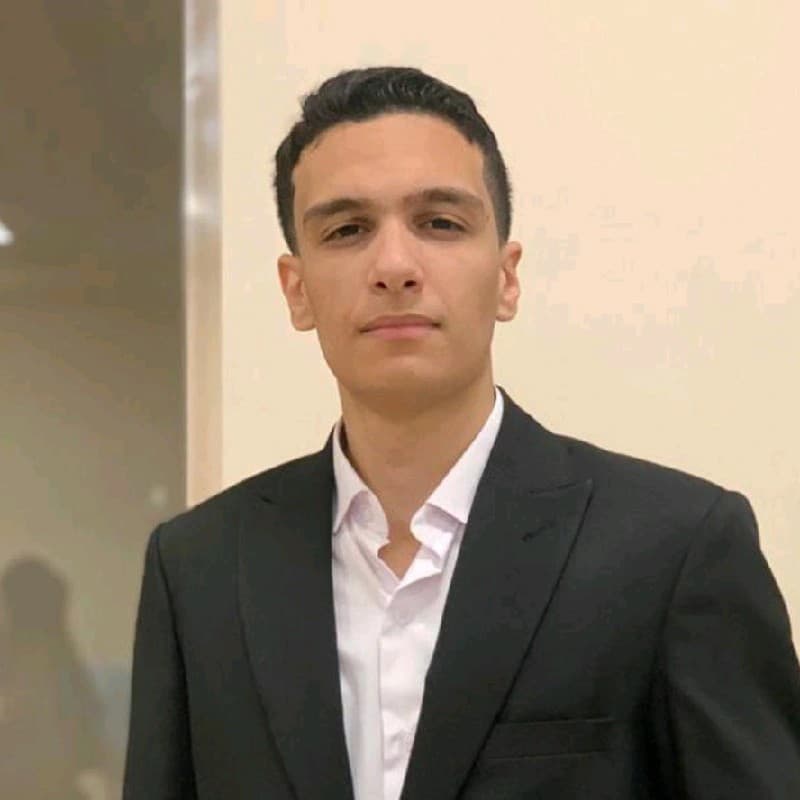
@yahiaelsayed19
created 4 months ago
export async function getStaticPaths() {
const paths = [{ params: { id: '1' } }, { params: { id: '2' } }];
return { paths, fallback: false };
}
export async function getStaticProps({ params }) {
return { props: { id: params.id } };
}
export default function Post({ id }) {
return <h1>Post ID: {id}</h1>;
}
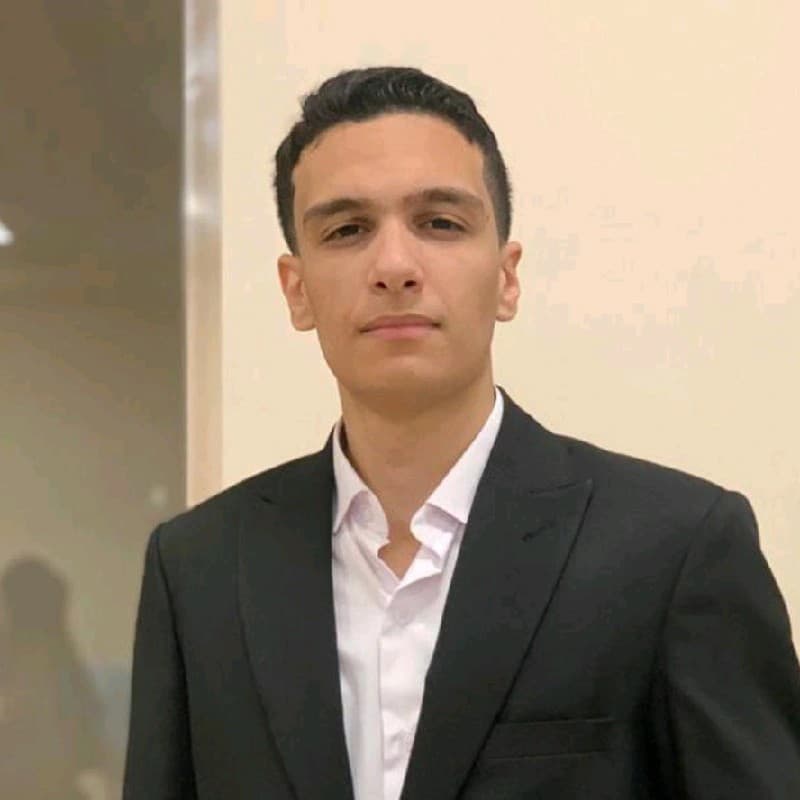
@yahiaelsayed19
created 4 months ago
export default function Home() {
return (
<div>
<h1>SSR Example</h1>
</div>
);
}
export async function getServerSideProps() {
return {
props: { message: 'This is SSR' },
};
}
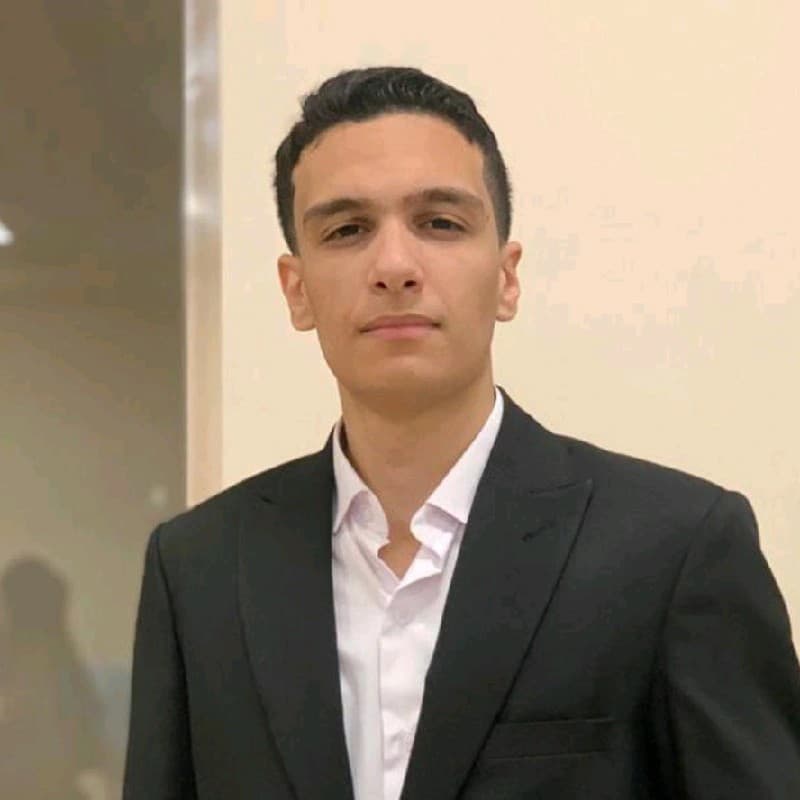
@yahiaelsayed19
created 4 months ago
import Link from 'next/link';
export default function Navbar() {
return (
<nav>
<Link href="/">Home</Link>
<Link href="/about">About</Link>
</nav>
);
}
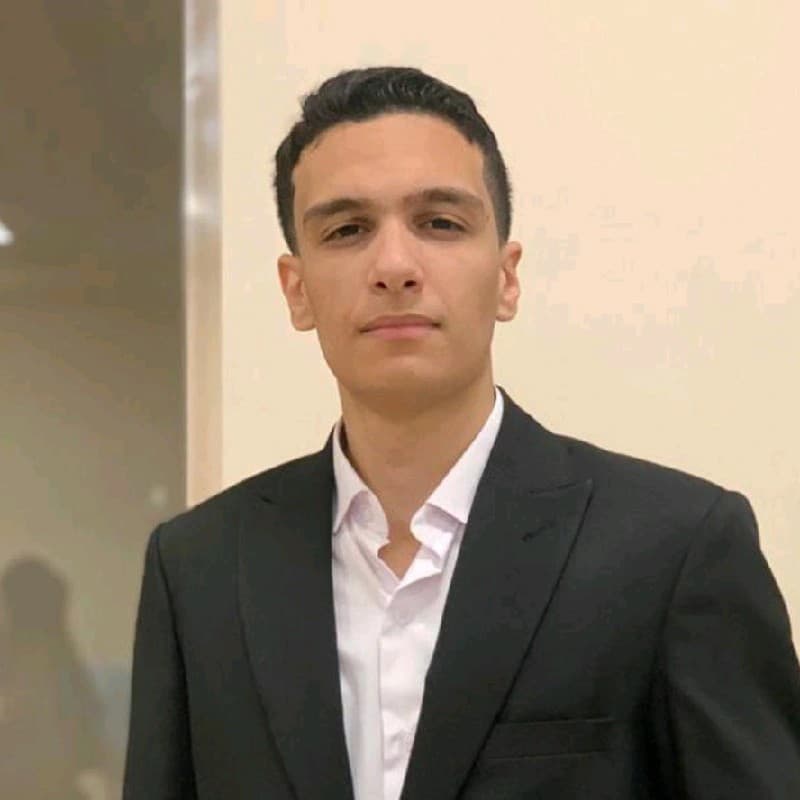
@yahiaelsayed19
created 4 months ago
import { useEffect, useState } from 'react';
export default function ClientSideData() {
const [data, setData] = useState(null);
useEffect(() => {
fetch('/api/hello')
.then(res => res.json())
.then(data => setData(data));
}, []);
return <h1>Data: {data ? data.message : 'Loading...'}</h1>;
}
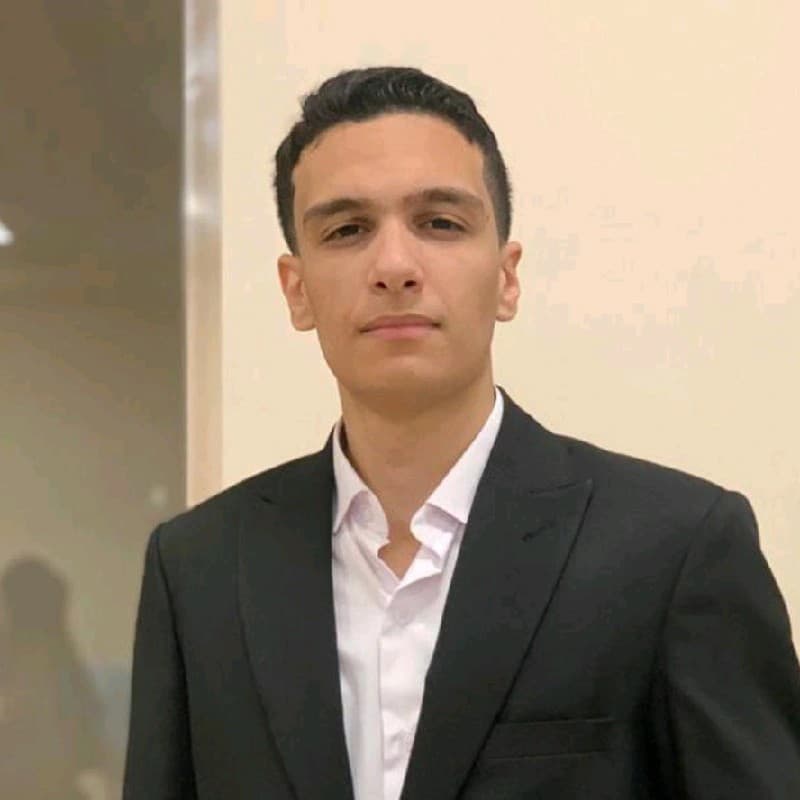
@yahiaelsayed19
created 4 months ago
import fs from 'fs';
import path from 'path';
export async function getStaticProps() {
const filePath = path.join(process.cwd(), 'data.json');
const fileContents = fs.readFileSync(filePath, 'utf8');
const data = JSON.parse(fileContents);
return { props: { data } };
}
export default function Data({ data }) {
return <h1>Data: {data.message}</h1>;
}
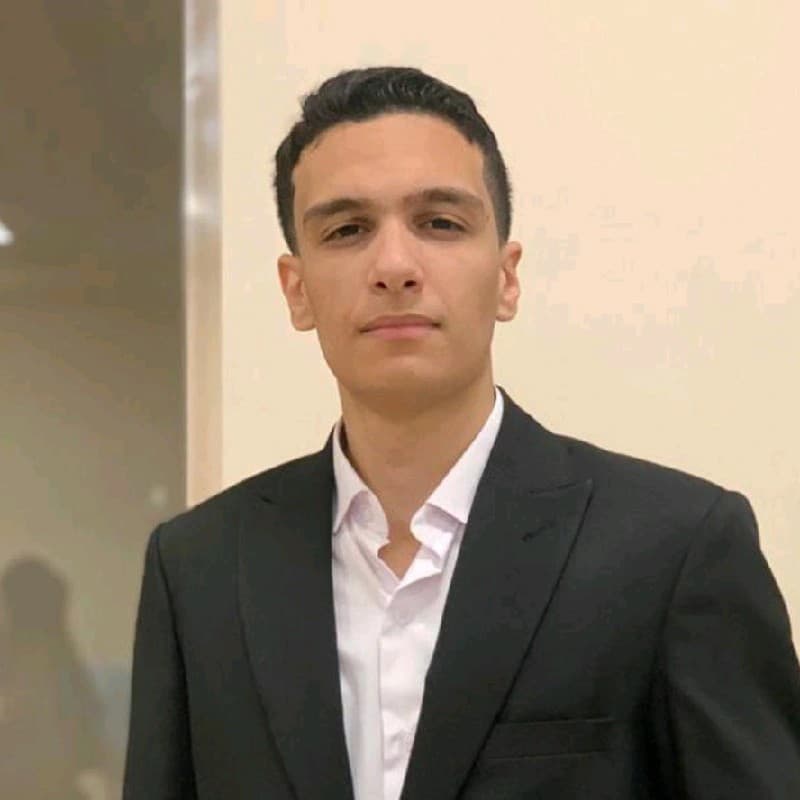
@yahiaelsayed19
created 4 months ago
import { useState } from 'react';
export default function Form() {
const [name, setName] = useState('');
const handleSubmit = (e) => {
e.preventDefault();
alert(`Hello, ${name}`);
};
return (
<form onSubmit={handleSubmit}>
<input type="text" value={name} onChange={(e) => setName(e.target.value)} />
<button type="submit">Submit</button>
</form>
);
}
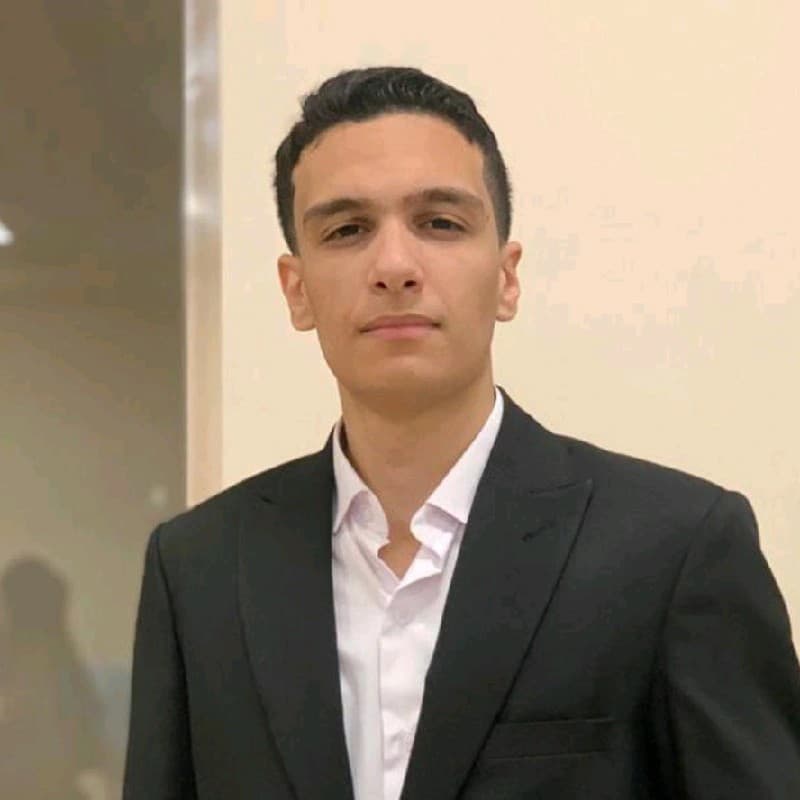
@yahiaelsayed19
created 4 months ago
import Link from 'next/link';
export default function Home() {
return (
<div>
<h1>Home</h1>
<Link href="/posts/first-post">Go to First Post</Link>
</div>
);
}
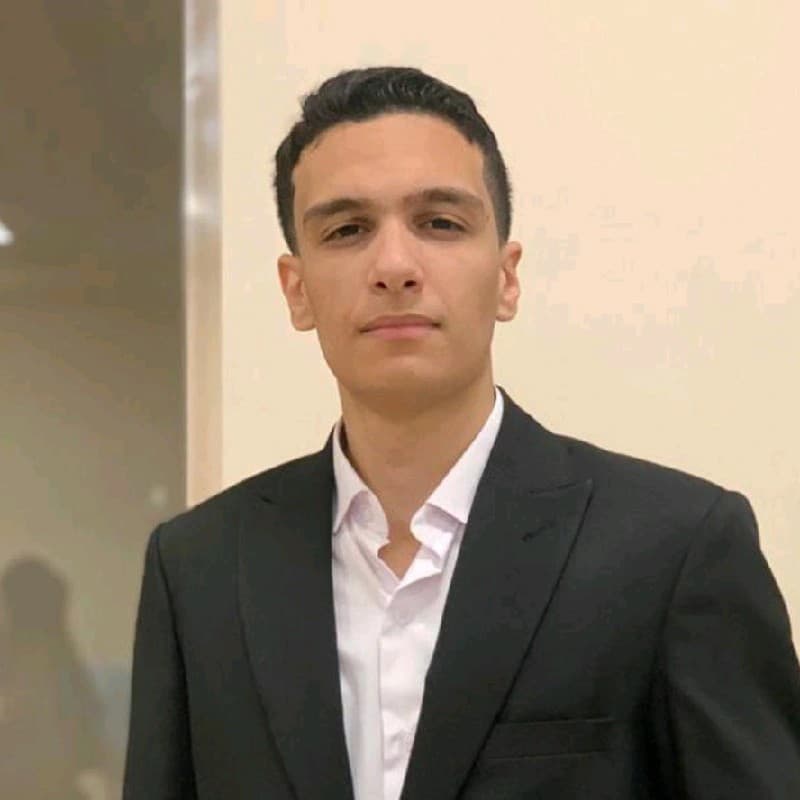
@yahiaelsayed19
created 4 months ago
export default function About() {
return <h1>About Us</h1>;
}
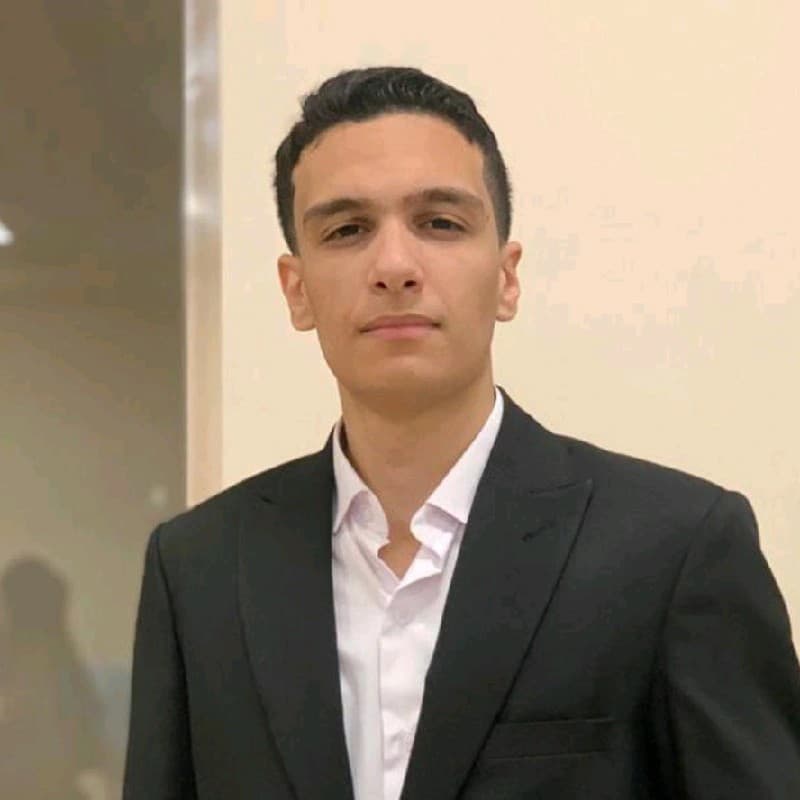
@yahiaelsayed19
created 4 months ago
import { useRouter } from 'next/router';
export default function CatchAll() {
const router = useRouter();
const { slug } = router.query;
return <h1>Slug: {slug?.join('/')}</h1>;
}
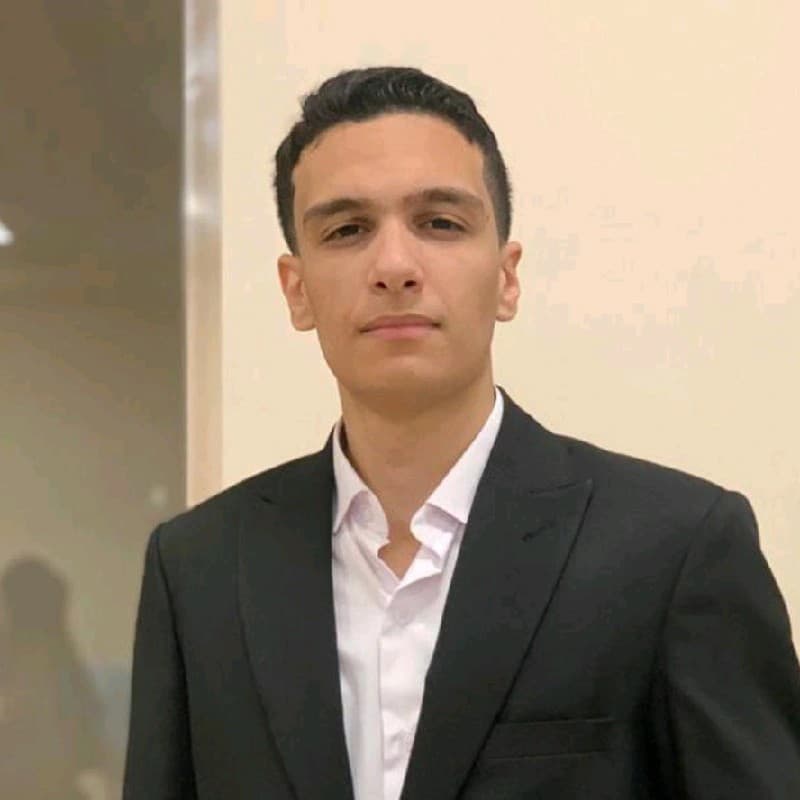
@yahiaelsayed19
created 3 months ago
import styles from './Home.module.css';
export default function Home() {
return <h1 className={styles.title}>Welcome to Next.js with CSS Modules</h1>;
}